Text-to-Speech API in C#
Looking for our Text to Speech Reader?
Featured In
In recent years, text-to-speech (TTS) technology has made significant strides, enabling a wide range of applications from accessibility tools to interactive voice response systems. As a software engineer with a penchant for making complex topics understandable, I’m excited to guide you through creating a text-to-speech application in C#. This tutorial will cover the essentials using Microsoft Azure's Cognitive Services and the .NET ecosystem, providing you with a solid foundation to build upon.
Getting Started with Text-to-Speech in C#
Prerequisites
Before we dive in, ensure you have the following set up:
- Visual Studio: An integrated development environment (IDE) for C#.
- .NET Core SDK: A cross-platform .NET implementation for building modern applications.
- Azure Subscription: Required to access Azure Cognitive Services.
- NuGet Package Manager: For managing dependencies in your project.
Step 1: Create a New .NET Core Console Application
Open Visual Studio and create a new .NET Core console application. Name your project something meaningful, like TextToSpeechDemo
.
bash
dotnet new console -n TextToSpeechDemo
cd TextToSpeechDemo
Step 2: Install Required NuGet Packages
Install the Microsoft.CognitiveServices.Speech
NuGet package, which contains the SDK for Azure Cognitive Services.
bash
dotnet add package Microsoft.CognitiveServices.Speech
Step 3: Set Up Azure Cognitive Services
- Create an Azure Cognitive Services Account: Navigate to the Azure portal and create a Cognitive Services account.
- Obtain API Keys: Once the account is created, get your API keys and endpoint URL.
Step 4: Write the Code
In your Program.cs
file, you’ll use the following namespaces:
csharp
using System;
using Microsoft.CognitiveServices.Speech;
Here’s a simple example to convert text to speech:
csharp
namespace TextToSpeechDemo
{
class Program
{
static async Task Main(string[] args)
{
var config = SpeechConfig.FromSubscription("YourSubscriptionKey", "YourServiceRegion");
using var synthesizer = new SpeechSynthesizer(config);
var result = await synthesizer.SpeakTextAsync("Hello, this is a text-to-speech demo using Azure Cognitive Services.");
if (result.Reason == ResultReason.SynthesizingAudioCompleted)
{
Console.WriteLine("Speech synthesized to speaker successfully.");
}
else if (result.Reason == ResultReason.Canceled)
{
var cancellation = SpeechSynthesisCancellationDetails.FromResult(result);
Console.WriteLine($"Error synthesizing. Reason: {cancellation.Reason}");
Console.WriteLine($"Error details: {cancellation.ErrorDetails}");
}
}
}
}
Replace "YourSubscriptionKey"
and "YourServiceRegion"
with your actual Azure Cognitive Services subscription key and service region.
Step 5: Run the Application
Run your application in Visual Studio. You should hear the text "Hello, this is a text-to-speech demo using Azure Cognitive Services." spoken aloud. This demonstrates the basic functionality of converting text to speech.
Step 6: Enhance the Application
Using SSML for Advanced Control
Speech Synthesis Markup Language (SSML) allows for more control over the speech output, such as voice, pitch, rate, and volume.
csharp
var ssml = @"
Hello, this is an SSML based text-to-speech synthesis.
";
var result = await synthesizer.SpeakSsmlAsync(ssml);
Saving Speech to an Audio File
You can also save the synthesized speech to an audio file.
csharp
var audioConfig = AudioConfig.FromWavFileOutput("output.wav");
using var synthesizer = new SpeechSynthesizer(config, audioConfig);
var result = await synthesizer.SpeakTextAsync("This text will be saved to an audio file.");
Exploring Other Options
Google Cloud Text-to-Speech
For those interested in exploring Google Cloud’s TTS, there are APIs available that provide similar functionality. You would need the Google.Cloud.TextToSpeech.V1
NuGet package and follow a similar setup process.
Open Source and Other Languages
There are open-source options and APIs available for other languages like Python, Java, and JavaScript. These can be useful for integrating TTS functionality into web applications or cross-platform software.
With the basics covered, you can now explore the vast potential of text-to-speech technology. Whether you’re building accessibility tools, creating interactive applications, or simply experimenting, TTS provides a powerful way to enhance user experiences.
For more detailed information, refer to the official Microsoft docs and explore GitHub repositories for more examples and advanced use cases.
Feel free to reach out if you have any questions or need further assistance. Happy coding!
Use the System.Speech.Synthesis
namespace in C# .NET on Windows to create a text-to-speech application, as shown in many beginner tutorials.
Some text-to-speech APIs offer free tiers with limited usage, such as the Google API and Microsoft Azure Cognitive Services.
Use Azure Cognitive Services or Google API for speech recognition in C# .NET, leveraging available SDKs and libraries for easy integration.
Develop a text-to-speech API using System.Speech.Synthesis
in ASP.NET for C# .NET applications, providing options for different voices, including female, and supporting various languagecode
settings.
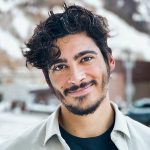
Cliff Weitzman
Cliff Weitzman is a dyslexia advocate and the CEO and founder of Speechify, the #1 text-to-speech app in the world, totaling over 100,000 5-star reviews and ranking first place in the App Store for the News & Magazines category. In 2017, Weitzman was named to the Forbes 30 under 30 list for his work making the internet more accessible to people with learning disabilities. Cliff Weitzman has been featured in EdSurge, Inc., PC Mag, Entrepreneur, Mashable, among other leading outlets.