Text to Speech API in Java: A Comprehensive Guide
Looking for our Text to Speech Reader?
Featured In
Text-to-speech (TTS) technology has revolutionized the way we interact with devices, enabling a seamless conversion of written text into spoken words. Whether it's for accessibility, convenience, or automation, TTS plays a vital role. In this article, I’ll walk you through how to implement text-to-speech functionality using Java, and explore various APIs and tools that can enhance your TTS projects.
Setting Up Your Java Environment
Before diving into the code, ensure you have Java installed on your machine. You can download the latest JDK from Oracle’s website. Once installed, you’re ready to start coding.
java
public class TextToSpeechExample {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
Using FreeTTS
FreeTTS is an open-source speech synthesis engine written in Java. It’s a good starting point for TTS in Java.
Adding FreeTTS Dependency
First, add the FreeTTS dependency to your Maven project:
xml
com.sun.speech
freetts
1.2.2
Basic Implementation
Here’s a simple implementation using FreeTTS:
java
import com.sun.speech.freetts.Voice;
import com.sun.speech.freetts.VoiceManager;
public class FreeTTSExample {
public static void main(String[] args) {
VoiceManager voiceManager = VoiceManager.getInstance();
Voice voice = voiceManager.getVoice("kevin16");
if (voice != null) {
voice.allocate();
voice.speak("Hello, this is a text-to-speech example using FreeTTS.");
voice.deallocate();
}
}
}
Setting Up Google Cloud
- Create a project on the Google Cloud Console.
- Enable the Google Cloud Text-to-Speech API.
- Create a service account and download the JSON key file.
Adding Google Cloud Dependency
Add the following dependency to your Maven project:
xml
com.google.cloud
google-cloud-texttospeech
1.0.0
Implementation
Here's how you can use the Google Cloud Text-to-Speech API in Java:
java
import com.google.cloud.texttospeech.v1.*;
import com.google.protobuf.ByteString;
import java.io.FileOutputStream;
import java.io.OutputStream;
public class GoogleTTSExample {
public static void main(String[] args) throws Exception {
try (TextToSpeechClient textToSpeechClient = TextToSpeechClient.create()) {
SynthesisInput input = SynthesisInput.newBuilder().setText("Hello, World!").build();
VoiceSelectionParams voice = VoiceSelectionParams.newBuilder()
.setLanguageCode("en-US")
.setSsmlGender(SsmlVoiceGender.NEUTRAL)
.build();
AudioConfig audioConfig = AudioConfig.newBuilder()
.setAudioEncoding(AudioEncoding.MP3)
.build();
SynthesizeSpeechResponse response = textToSpeechClient.synthesizeSpeech(input, voice, audioConfig);
ByteString audioContents = response.getAudioContent();
try (OutputStream out = new FileOutputStream("output.mp3")) {
out.write(audioContents.toByteArray());
}
}
}
}
Exploring Other TTS Options
Microsoft Azure Cognitive Services
Microsoft’s Text-to-Speech service offers a robust SDK with support for multiple languages and voices. You can find detailed documentation and examples on their official docs.
IBM Watson Text to Speech
IBM Watson provides a powerful TTS API with advanced speech synthesis capabilities. Visit the IBM Watson documentation for setup instructions and code samples.
OpenAI and Other APIs
OpenAI and other AI services offer state-of-the-art machine learning models that can be integrated for advanced TTS functionalities. While OpenAI itself doesn’t provide a direct TTS API, combining their language models with other TTS engines can yield impressive results.
Try Speechify Text to Speech API
The Speechify Text to Speech API is a powerful tool designed to convert written text into spoken words, enhancing accessibility and user experience across various applications. It leverages advanced speech synthesis technology to deliver natural-sounding voices in multiple languages, making it an ideal solution for developers looking to implement audio reading features in apps, websites, and e-learning platforms.
With its easy-to-use API, Speechify enables seamless integration and customization, allowing for a wide range of applications from reading aids for the visually impaired to interactive voice response systems.
TTS Beyond Java
Using Python
Python is popular for TTS due to its simplicity and the extensive libraries available, such as gTTS and pyttsx3.
Node.js and JavaScript
Node.js, with libraries like say.js
, offers easy integration for TTS in web applications.
PHP and Other Languages
PHP developers can use libraries like php-google-cloud-tts
to add TTS functionality to their projects.
Implementing text-to-speech in Java opens up numerous possibilities for creating interactive and accessible applications. Whether you’re using open-source tools like FreeTTS or leveraging the power of cloud services from Google, Microsoft, or IBM, the potential is vast. Explore different APIs, experiment with various voices and languages, and integrate TTS to enhance your applications’ user experience.
For more detailed examples and code snippets, visit the GitHub repository and explore the official documentation of each service mentioned.
Happy coding!
Some text-to-speech APIs, like Google Cloud's TTS, offer free tiers with usage limits, while others may require a subscription for full access. Check the specific API's pricing details for more information.
The Java Speech API (JSAPI), specifically javax.speech
, provides a framework for speech recognition and synthesis in Java applications on Windows and other platforms.
To create a text-to-speech API, you can use a synthesizer like FreeTTS or integrate cloud-based services such as Google Cloud Text-to-Speech API, ensuring proper encoding and outputting the audio file in the desired format.
To install the Java Speech API (JSAPI), download the necessary javax.speech
libraries, include them in your project, and configure your development environment as per the tutorial guidelines for platforms like Windows and Android.
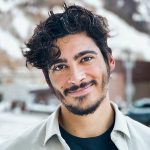
Cliff Weitzman
Cliff Weitzman is a dyslexia advocate and the CEO and founder of Speechify, the #1 text-to-speech app in the world, totaling over 100,000 5-star reviews and ranking first place in the App Store for the News & Magazines category. In 2017, Weitzman was named to the Forbes 30 under 30 list for his work making the internet more accessible to people with learning disabilities. Cliff Weitzman has been featured in EdSurge, Inc., PC Mag, Entrepreneur, Mashable, among other leading outlets.