Text to Speech API in Golang: A Comprehensive Guide
Looking for our Text to Speech Reader?
Featured In
Hey there! If you're looking to create a Text-to-Speech (TTS) application using Golang, you've come to the right place. This tutorial will guide you through the process step-by-step, from setting up your environment to synthesizing speech. We'll leverage Google Cloud's Text-to-Speech API and the Go programming language to achieve this. Let's dive in!
Prerequisites
Before we start, make sure you have the following:
- Golang installed: You can download it from the official Go website.
- Google Cloud account: Sign up if you haven't already, and create a new project.
- API key: Enable the Cloud Text-to-Speech API and generate an API key.
Setting Up Your Project
First, let's set up our Go project. Create a new directory for your project and navigate into it:
bash
mkdir tts-go
cd tts-go
Initialize a new Go module:
bash
go mod init tts-go
Next, we need to install the necessary client libraries. Run the following command to get the Google Cloud Text-to-Speech client library:
bash
go get -u cloud.google.com/go/texttospeech/apiv1
We'll also need the following dependencies:
bash
go get -u google.golang.org/genproto/googleapis/cloud/texttospeech/v1
go get -u google.golang.org/grpc
Writing the Code
Now, let's create a file named main.go
and start coding. This is where the magic happens!
go
package main
import (
"context"
"fmt"
"io/ioutil"
"log"
"os"
texttospeech "cloud.google.com/go/texttospeech/apiv1"
texttospeechpb "google.golang.org/genproto/googleapis/cloud/texttospeech/v1"
)
func main() {
ctx := context.Background()
// Creates a client.
client, err := texttospeech.NewClient(ctx)
if err != nil {
log.Fatal(err)
}
defer client.Close()
// The text input to be synthesized.
req := &texttospeechpb.SynthesizeSpeechRequest{
Input: &texttospeechpb.SynthesisInput{
InputSource: &texttospeechpb.SynthesisInput_Text{Text: "Hello, world!"},
},
// Build the voice request; select the language code ("en-US") and the SSML voice gender ("neutral").
Voice: &texttospeechpb.VoiceSelectionParams{
LanguageCode: "en-US",
SsmlGender: texttospeechpb.SsmlVoiceGender_NEUTRAL,
},
// Select the type of audio file you want returned.
AudioConfig: &texttospeechpb.AudioConfig{
AudioEncoding: texttospeechpb.AudioEncoding_LINEAR16,
},
}
// Performs the text-to-speech request on the text input with the selected voice parameters and audio file type.
resp, err := client.SynthesizeSpeech(ctx, req)
if err != nil {
log.Fatal(err)
}
// The resp's AudioContent is binary.
filename := "output.wav"
err = ioutil.WriteFile(filename, resp.AudioContent, 0644)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Audio content written to file: %v\n", filename)
}
Explanation
- Imports: We import necessary packages, including the Google Cloud Text-to-Speech client library and context package.
- Client Initialization: We create a new client using the
texttospeech.NewClient(ctx)
function. - Request Setup: We build the request with the text to be synthesized, voice parameters, and audio configuration.
- Synthesis: We call
client.SynthesizeSpeech(ctx, req)
to perform the text-to-speech synthesis. - Write Audio: We write the audio content to an output file named
output.wav
.
Running the Code
Make sure your GOOGLE_APPLICATION_CREDENTIALS
environment variable is set to the path of your service account key file. Then, run the code:
bash
go run main.go
You should see an output file named output.wav
in your project directory containing the synthesized speech.
Additional Resources
If you're interested in more use cases or advanced configurations, check out the official [Google Cloud Text-to-Speech API docs. You can also explore open-source libraries like Hegedustibor for offline TTS capabilities.
Congratulations! You've just created a simple Text-to-Speech application using Golang and Google Cloud's Text-to-Speech API. This is just the beginning—there's so much more you can do with TTS, from building interactive voice applications to generating audio content dynamically. Happy coding!
If you have any questions or need further assistance, feel free to ask. Until next time, happy coding!
Try Speechify Text to Speech API
The Speechify Text to Speech API is a powerful tool designed to convert written text into spoken words, enhancing accessibility and user experience across various applications. It leverages advanced speech synthesis technology to deliver natural-sounding voices in multiple languages, making it an ideal solution for developers looking to implement audio reading features in apps, websites, and e-learning platforms.
With its easy-to-use API, Speechify enables seamless integration and customization, allowing for a wide range of applications from reading aids for the visually impaired to interactive voice response systems.
Google TTS API offers a free tier with limited usage, but for higher usage, pricing applies based on the volume of audio data processed.
Google Cloud Text-to-Speech API is highly realistic, utilizing advanced deep learning models to convert text into natural-sounding speech in multiple languages, including English.
To create a Text-to-Speech API, you need to set up a server that takes text input, uses a TTS SDK or library to convert text to audio data, and returns the audio file.
To get a Google TTS API key, enable the API in Google Cloud Console, go to the API & Services section, and create a new API key under the credentials tab.
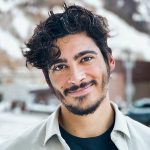
Cliff Weitzman
Cliff Weitzman is a dyslexia advocate and the CEO and founder of Speechify, the #1 text-to-speech app in the world, totaling over 100,000 5-star reviews and ranking first place in the App Store for the News & Magazines category. In 2017, Weitzman was named to the Forbes 30 under 30 list for his work making the internet more accessible to people with learning disabilities. Cliff Weitzman has been featured in EdSurge, Inc., PC Mag, Entrepreneur, Mashable, among other leading outlets.