Text to Speech API in Kotlin
Looking for our Text to Speech Reader?
Featured In
Creating an Android application with text-to-speech (TTS) functionality can be a fascinating project, especially for beginners. In this tutorial, I'll guide you through integrating a TTS API in an Android app using Kotlin. We'll cover the essentials, from setting up your project in Android Studio to implementing TTS features.
Getting Started
Prerequisites
Before we dive in, ensure you have the following installed:
- Android Studio: The primary IDE for Android development.
- Kotlin: The preferred language for modern Android apps.
- Java: Necessary for certain Android functionalities.
- Android SDK: Essential for building and testing your app.
Creating a New Project
- Open Android Studio and create a new project.
- Choose an Empty Activity template.
- Name your project
TTSApp
, select Kotlin as the language, and finish the setup.
Setting Up the Layout
In your activity_main.xml
file, define the UI components:
xml
Adding Text-to-Speech Functionality
First, add the necessary imports in your MainActivity.kt
file:
kotlin
import android.os.Bundle
import android.speech.tts.TextToSpeech
import android.widget.Button
import android.widget.EditText
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import java.util.Locale
Next, implement the TextToSpeech.OnInitListener
interface and initialize the TTS engine:
kotlin
class MainActivity : AppCompatActivity(), TextToSpeech.OnInitListener {
private lateinit var tts: TextToSpeech
private lateinit var editText: EditText
private lateinit var btnSpeak: Button
private lateinit var textView: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
editText = findViewById(R.id.editText)
btnSpeak = findViewById(R.id.btnSpeak)
textView = findViewById(R.id.textView)
tts = TextToSpeech(this, this)
btnSpeak.setOnClickListener {
speakOut()
}
}
override fun onInit(status: Int) {
if (status == TextToSpeech.SUCCESS) {
val result = tts.setLanguage(Locale.US)
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
textView.text = "Language not supported"
} else {
btnSpeak.isEnabled = true
}
} else {
textView.text = "Initialization failed"
}
}
private fun speakOut() {
val text = editText.text.toString()
tts.speak(text, TextToSpeech.QUEUE_FLUSH, null, "")
}
override fun onDestroy() {
if (tts != null) {
tts.stop()
tts.shutdown()
}
super.onDestroy()
}
}
Customizing TTS
You can adjust the speech rate and pitch to make the TTS sound more natural:
kotlin
tts.setSpeechRate(1.0f) // Normal speed
tts.setPitch(1.0f) // Normal pitch
Handling Edge Cases
Ensure you handle scenarios where TTS might not be available or supported:
kotlin
override fun onInit(status: Int) {
if (status == TextToSpeech.SUCCESS) {
val result = tts.setLanguage(Locale.US)
if (result == TextToSpeech.LANG_MISSING_DATA || result == TextToSpeech.LANG_NOT_SUPPORTED) {
textView.text = "Language not supported"
} else {
btnSpeak.isEnabled = true
}
} else {
textView.text = "Initialization failed"
}
}
Additional Features
Notifications
You can use TTS to read out notifications, enhancing accessibility. Implement a notification listener to achieve this.
Widgets
Create a widget that triggers TTS when clicked. Use setOnClickListener
to handle click events and findViewById
to link UI elements.
Integration with Other APIs
Integrate with Speech Recognition APIs to convert speech to text and then read it out using TTS. You can also use libraries like Retrofit for network calls or Firebase for cloud functionalities.
Advanced Topics
- Jetpack Compose: For a modern UI toolkit, consider migrating to Jetpack Compose.
- Text-to-Speech in Flutter: If you prefer cross-platform development, Flutter offers TTS plugins.
- Python for Backend: Use Python for backend services that can send text to your Android app for TTS.
- JavaScript Integration: Use JavaScript for web-based TTS applications.
- GitHub Repositories: Explore GitHub for sample projects and libraries.
In this tutorial, we explored the basics of integrating a Text-to-Speech API in an Android application using Kotlin. We covered setting up the project, adding TTS functionality, and handling edge cases. TTS is a powerful tool for creating more accessible and interactive Android apps. Happy coding!
Feel free to share your thoughts or questions in the comments. If you run into any issues, I'll be glad to help out.
Speechify Text to Speech API
The Speechify Text to Speech API is a powerful tool designed to convert written text into spoken words, enhancing accessibility and user experience across various applications. It leverages advanced speech synthesis technology to deliver natural-sounding voices in multiple languages, making it an ideal solution for developers looking to implement audio reading features in apps, websites, and e-learning platforms.
With its easy-to-use API, Speechify enables seamless integration and customization, allowing for a wide range of applications from reading aids for the visually impaired to interactive voice response systems.
To implement text-to-speech in Android Kotlin, initialize the TextToSpeech
engine in your MainActivity
and use the speak
method to synthesize speech from text.
The text-to-speech API in Android allows your app to convert text to spoken words using the device's text-to-speech engine.
Add text-to-speech in your Android app by integrating the TextToSpeech
engine in Kotlin, handling initialization with TextToSpeech.OnInitListener
, and using speak
for playback.
The TTS engine in Android supports multiple languages, including English, Spanish, and Chinese, with the availability depending on the installed language packs.
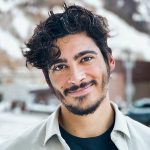
Cliff Weitzman
Cliff Weitzman is a dyslexia advocate and the CEO and founder of Speechify, the #1 text-to-speech app in the world, totaling over 100,000 5-star reviews and ranking first place in the App Store for the News & Magazines category. In 2017, Weitzman was named to the Forbes 30 under 30 list for his work making the internet more accessible to people with learning disabilities. Cliff Weitzman has been featured in EdSurge, Inc., PC Mag, Entrepreneur, Mashable, among other leading outlets.